Unlocking Smooth UI Updates in Flutter with watch_it
Author
Tomislav Stipanić
Date Published
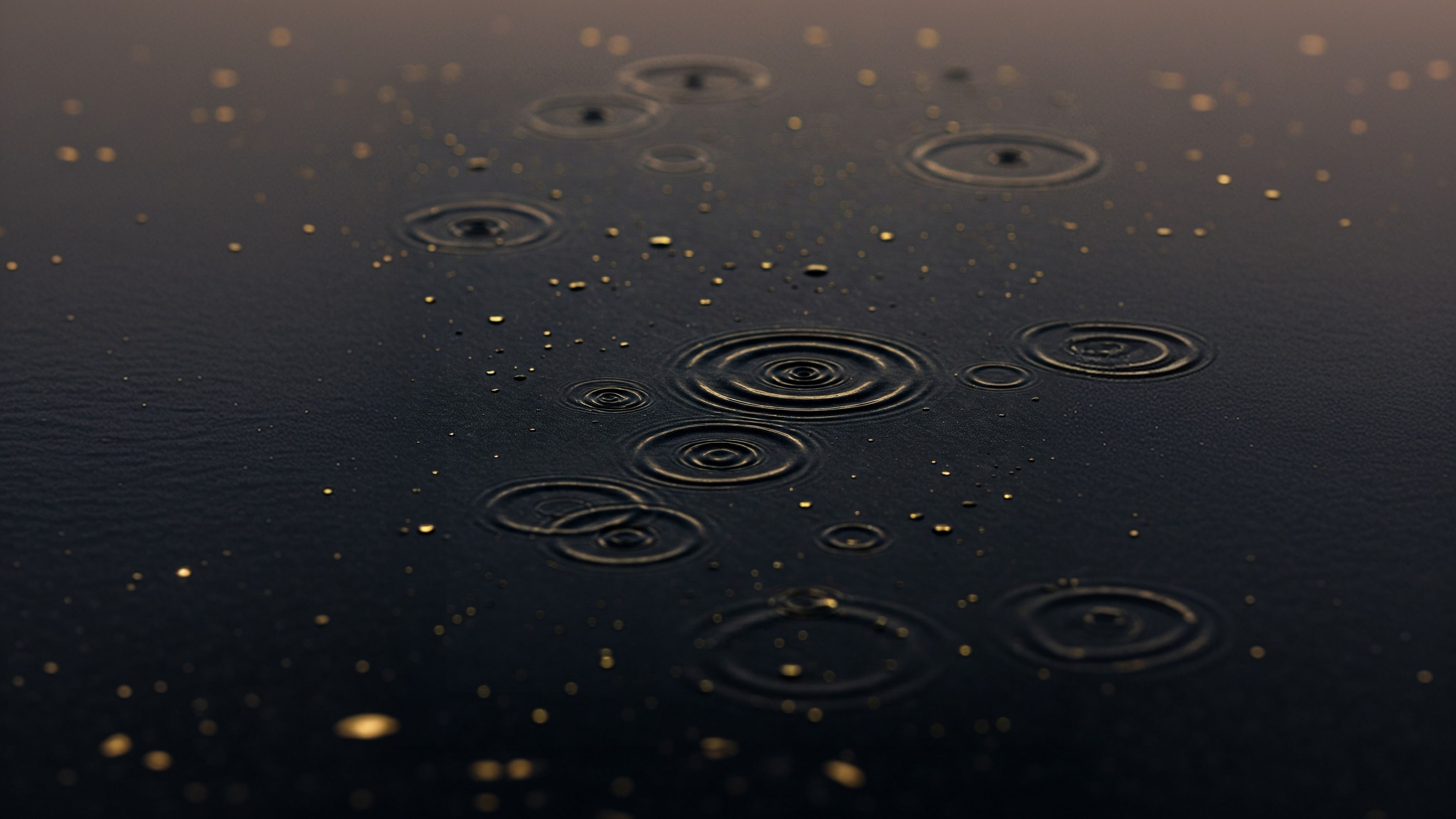
Managing state in Flutter can sometimes feel overwhelming, especially with the variety of approaches available. watch_it
is a lightweight and efficient solution built on top of get_it
, designed to simplify state management by enabling automatic widget rebuilding without excessive boilerplate.
Why Consider watch_it?
Automatic UI Updates – No more manual setState()
calls. watch_it automatically rebuilds widgets when observed data changes, keeping your UI in sync with minimal effort.
Seamless Integration with get_it – If you're already using get_it for dependency injection, adding watch_it is almost effortless. It works hand in hand, making state management smooth and intuitive.
Lightweight and Minimalist – Unlike heavier state management solutions like provider or bloc, watch_it keeps things clean and simple. No unnecessary boilerplate, just efficient state management.
No Manual Listener Handling – Forget about adding and removing listeners manually. watch_it observes properties and updates UI components automatically.
Flexible State Management Options – Works with various data sources, including
Listenable, ValueListenable, Stream and Future
This flexibility means you can pick the best approach for your use case without being locked into a rigid framework.
Easier Dependency Access – watch_it makes it simple to access dependencies across your app, reducing the need for prop drilling.
Encourages Clean Architecture – Since it's built on get_it, watch_it naturally promotes a well-structured separation between UI and business logic.
Active Development & Community Support – The package continues to receive updates and is gaining traction within the Flutter community.
watch_it provides a set of watch
functions to observe various data types:
watch
: Observes any Listenable object.watchIt
: Observes any Listenable registered in get_it.watchValue
: Observes a ValueListenable property of an object in get_it.watchPropertyValue
: Observes a specific property of a Listenable object and rebuilds only when the property value changes.watchStream
: Observes a Stream and rebuilds when the Stream emits a new value.watchFuture
: Observes a Future and rebuilds when the Future completes.
How to Use watch_it in Your Flutter Project
Step 1: Install the Package
Add watch_it to your pubspec.yaml
:
1dependencies:2 watch_it: ^1.6.2
Then, run: flutter pub get
Step 2: Register Your Services
Example of a UserModel:
1class UserModel with ChangeNotifier {2 String _name = "Guest";34 String get name => _name;56 void updateName(String newName) {7 _name = newName;8 notifyListeners();9 }10}1112void setupLocator() {13 di.registerSingleton<UserModel>(UserModel());14}
Step 3: Watch and Rebuild Widgets
Create a widget that updates when UserModel changes:
1class UserNameText extends WatchingWidget {2 @override3 Widget build(BuildContext context) {4 final userName = watchPropertyValue((UserModel model) => model.name);5 return Text("Hello, $userName!");6 }7}
Now, the widget rebuilds whenever UserModel updates—without extra state management code.
Conclusion
If you need a straightforward and effective state management approach, especially when using get_it, watch_it is a great choice. It keeps code manageable, improves UI responsiveness, and reduces unnecessary complexity.